mirror of
https://github.com/ChrisTitusTech/winutil.git
synced 2025-08-07 20:46:22 -05:00
Compile Winutil
This commit is contained in:
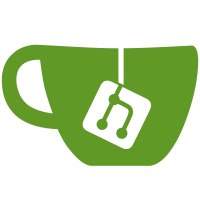
committed by
github-actions[bot]
![github-actions[bot]](/assets/img/avatar_default.png)
parent
ce7d14b227
commit
47dbbfb4ff
91
winutil.ps1
91
winutil.ps1
@ -66,28 +66,94 @@ function ConvertTo-Icon {
|
||||
<#
|
||||
|
||||
.DESCRIPTION
|
||||
This function will convert PNG to ICO file
|
||||
This function will convert BMP, GIF, EXIF, JPG, PNG and TIFF to ICO file
|
||||
|
||||
.PARAMETER bitmapPath
|
||||
The file path to bitmap image to make '.ico' file out of.
|
||||
Supported file types according to Microsoft Documentation is the following:
|
||||
BMP, GIF, EXIF, JPG, PNG and TIFF.
|
||||
|
||||
.PARAMETER iconPath
|
||||
The file path to write the new '.ico' resource.
|
||||
|
||||
.PARAMETER overrideIconFile
|
||||
An optional boolean Parameter that makes the function overrides
|
||||
the Icon File Path if the file exists. Defaults to $true.
|
||||
|
||||
.EXAMPLE
|
||||
ConvertTo-Icon -bitmapPath "$env:TEMP\cttlogo.png" -iconPath $iconPath
|
||||
try {
|
||||
ConvertTo-Icon -bitmapPath "$env:TEMP\cttlogo.png" -iconPath "$env:TEMP\cttlogo.ico"
|
||||
} catch [System.IO.FileNotFoundException] {
|
||||
# Handle the thrown exception here...
|
||||
}
|
||||
|
||||
This Example makes a '.ico' file at "$env:TEMP\cttlogo.ico" File Path using the bitmap file
|
||||
found in "$env:TEMP\cttlogo.png", the function overrides the '.ico' File if it's found.
|
||||
this function will throw a FileNotFound Exception at the event of not finding the provided bitmap File Path.
|
||||
|
||||
.EXAMPLE
|
||||
try {
|
||||
ConvertTo-Icon "$env:TEMP\cttlogo.png" "$env:TEMP\cttlogo.ico"
|
||||
} catch [System.IO.FileNotFoundException] {
|
||||
# Handle the thrown exception here...
|
||||
}
|
||||
|
||||
This Example is the same as Example 1, but uses Positional Parameters instead.
|
||||
|
||||
.EXAMPLE
|
||||
if (Test-Path "$env:TEMP\cttlogo.png") {
|
||||
ConvertTo-Icon -bitmapPath "$env:TEMP\cttlogo.png" -iconPath "$env:TEMP\cttlogo.ico"
|
||||
}
|
||||
|
||||
This Example is same as Example 1, but checks if the bitmap File exists before calling 'ConvertTo-Icon' Function.
|
||||
This's the recommended way of using this function, as it doesn't require any try-catch blocks.
|
||||
|
||||
.EXAMPLE
|
||||
try {
|
||||
ConvertTo-Icon -bitmapPath "$env:TEMP\cttlogo.png" -iconPath "$env:TEMP\cttlogo.ico" -overrideIconFile $false
|
||||
} catch [System.IO.FileNotFoundException] {
|
||||
# Handle the thrown exception here...
|
||||
}
|
||||
|
||||
This Example make use of '-overrideIconFile' Optional Parameter, the default for this paramter is $true.
|
||||
By doing '-overrideIconFile $false', the 'ConvertTo-Icon' function will raise an exception that needs to be catched throw a 'catch' Code Block,
|
||||
otherwise it'll crash the running PowerShell instance/process.
|
||||
|
||||
#>
|
||||
param( [Parameter(Mandatory=$true)]
|
||||
$bitmapPath,
|
||||
$iconPath = "$env:temp\newicon.ico"
|
||||
param(
|
||||
[Parameter(Mandatory=$true, position=0)]
|
||||
[string]$bitmapPath,
|
||||
[Parameter(Mandatory=$true, position=1)]
|
||||
[string]$iconPath,
|
||||
[Parameter(position=2)]
|
||||
[bool]$overrideIconFile = $true
|
||||
)
|
||||
|
||||
Add-Type -AssemblyName System.Drawing
|
||||
|
||||
if (Test-Path $bitmapPath) {
|
||||
if ((Test-Path $iconPath) -AND ($overrideIconFile -eq $false)) {
|
||||
Write-Host "[ConvertTo-Icon] Icon File is found at '$iconPath', and the 'overrideIconFile' Parameter is set to '$overrideIconFile'. Skipping the bitmap to icon convertion..." -ForegroundColor Yellow
|
||||
return
|
||||
}
|
||||
|
||||
# Load bitmap file into memory, and make an Icon version out of it
|
||||
$b = [System.Drawing.Bitmap]::FromFile($bitmapPath)
|
||||
$icon = [System.Drawing.Icon]::FromHandle($b.GetHicon())
|
||||
|
||||
# Create the folder for the new icon file if it doesn't exists
|
||||
$iconFolder = (New-Object System.IO.FileInfo($iconPath)).Directory.FullName
|
||||
[System.IO.Directory]::CreateDirectory($iconFolder) | Out-Null
|
||||
|
||||
# Write the Icon File and do some cleaning-up
|
||||
$file = New-Object System.IO.FileStream($iconPath, 'OpenOrCreate')
|
||||
$icon.Save($file)
|
||||
$file.Close()
|
||||
$icon.Dispose()
|
||||
#explorer "/SELECT,$iconpath"
|
||||
}
|
||||
else { Write-Warning "$BitmapPath does not exist" }
|
||||
else {
|
||||
throw [System.IO.FileNotFoundException] "[ConvertTo-Icon] The provided bitmap File Path is not found at '$bitmapPath'."
|
||||
}
|
||||
}
|
||||
function Copy-Files {
|
||||
<#
|
||||
@ -4808,8 +4874,10 @@ function Invoke-WPFShortcut {
|
||||
$ArgumentsToSourceExe = "$powershell '$IRM'"
|
||||
$DestinationName = "WinUtil.lnk"
|
||||
|
||||
Invoke-WebRequest -Uri "https://christitus.com/images/logo-full.png" -OutFile "$env:TEMP\cttlogo.png"
|
||||
|
||||
if (Test-Path -Path "$env:TEMP\cttlogo.png") {
|
||||
$iconPath = "$env:SystempRoot\cttlogo.ico"
|
||||
$iconPath = "$env:LOCALAPPDATA\winutil\cttlogo.ico"
|
||||
ConvertTo-Icon -bitmapPath "$env:TEMP\cttlogo.png" -iconPath $iconPath
|
||||
}
|
||||
}
|
||||
@ -4821,20 +4889,23 @@ function Invoke-WPFShortcut {
|
||||
$FileBrowser.Filter = "Shortcut Files (*.lnk)|*.lnk"
|
||||
$FileBrowser.FileName = $DestinationName
|
||||
|
||||
# Do an Early Return if The Save Shortcut operation was cancel by User's Input.
|
||||
# Do an Early Return if the Save Operation was canceled by User's Input.
|
||||
$FileBrowserResult = $FileBrowser.ShowDialog()
|
||||
$DialogResultEnum = New-Object System.Windows.Forms.DialogResult
|
||||
if (-not ($FileBrowserResult -eq $DialogResultEnum::OK)) {
|
||||
return
|
||||
}
|
||||
|
||||
# Prepare the Shortcut paramter
|
||||
$WshShell = New-Object -comObject WScript.Shell
|
||||
$Shortcut = $WshShell.CreateShortcut($FileBrowser.FileName)
|
||||
$Shortcut.TargetPath = $SourceExe
|
||||
$Shortcut.Arguments = $ArgumentsToSourceExe
|
||||
if ($null -ne $iconPath) {
|
||||
if ($iconPath -ne $null) {
|
||||
$shortcut.IconLocation = $iconPath
|
||||
}
|
||||
|
||||
# Save the Shortcut to disk
|
||||
$Shortcut.Save()
|
||||
|
||||
if ($RunAsAdmin -eq $true) {
|
||||
|
Reference in New Issue
Block a user