mirror of
https://github.com/ChrisTitusTech/winutil.git
synced 2024-11-15 07:05:51 -06:00
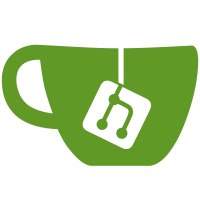
* enhance asset mgmt - invoke ico directly - invoke ico only at shortcut creation - remove "ConvertTo-Icon" Function file - removed image from xaml - added stackpanel to xaml - added functions to create viewbox with image - added logic to add image via code to xaml & customdialog - changed title color for customdialog - remove webinvokes for assets from main.ps1 TODO: convert images into bitmap base64 string & add them directly into invoke-WinUtiltaskbaritem.ps1 * improve viewboxfunction - add logo + checkmark + warning - add params - render on param "render" - custom dialog logo function call fix - main logo function call fix - update winutiltaskbaritem to use new images * fix sizing - warning & checkmark sizing fix - remove unneded comments * fixes - remove unneeded redundancy of "$canvas = New-Object Windows.Controls.Canvas" - adjust empty lines - use LimeGreen instead of Green * fix layouting * fixes - use correct ctt blue color #0567ff - remove unneeded comments - rename Logoview to assets * performance improvement instead of rendering the image another time on each item switch, it caches it at the start and uses the render afterwards * redo gray part of winutil logo * correct gray shade * fix coloring * ise ico if available --------- Co-authored-by: Chris Titus <contact@christitus.com>
87 lines
3.0 KiB
PowerShell
87 lines
3.0 KiB
PowerShell
function Set-WinUtilTaskbaritem {
|
|
<#
|
|
|
|
.SYNOPSIS
|
|
Modifies the Taskbaritem of the WPF Form
|
|
|
|
.PARAMETER value
|
|
Value can be between 0 and 1, 0 being no progress done yet and 1 being fully completed
|
|
Value does not affect item without setting the state to 'Normal', 'Error' or 'Paused'
|
|
Set-WinUtilTaskbaritem -value 0.5
|
|
|
|
.PARAMETER state
|
|
State can be 'None' > No progress, 'Indeterminate' > inf. loading gray, 'Normal' > Gray, 'Error' > Red, 'Paused' > Yellow
|
|
no value needed:
|
|
- Set-WinUtilTaskbaritem -state "None"
|
|
- Set-WinUtilTaskbaritem -state "Indeterminate"
|
|
value needed:
|
|
- Set-WinUtilTaskbaritem -state "Error"
|
|
- Set-WinUtilTaskbaritem -state "Normal"
|
|
- Set-WinUtilTaskbaritem -state "Paused"
|
|
|
|
.PARAMETER overlay
|
|
Overlay icon to display on the taskbar item, there are the presets 'None', 'logo' and 'checkmark' or you can specify a path/link to an image file.
|
|
CTT logo preset:
|
|
- Set-WinUtilTaskbaritem -overlay "logo"
|
|
Checkmark preset:
|
|
- Set-WinUtilTaskbaritem -overlay "checkmark"
|
|
Warning preset:
|
|
- Set-WinUtilTaskbaritem -overlay "warning"
|
|
No overlay:
|
|
- Set-WinUtilTaskbaritem -overlay "None"
|
|
Custom icon (needs to be supported by WPF):
|
|
- Set-WinUtilTaskbaritem -overlay "C:\path\to\icon.png"
|
|
|
|
.PARAMETER description
|
|
Description to display on the taskbar item preview
|
|
Set-WinUtilTaskbaritem -description "This is a description"
|
|
#>
|
|
param (
|
|
[string]$state,
|
|
[double]$value,
|
|
[string]$overlay,
|
|
[string]$description
|
|
)
|
|
|
|
if ($value) {
|
|
$sync["Form"].taskbarItemInfo.ProgressValue = $value
|
|
}
|
|
|
|
if ($state) {
|
|
switch ($state) {
|
|
'None' { $sync["Form"].taskbarItemInfo.ProgressState = "None" }
|
|
'Indeterminate' { $sync["Form"].taskbarItemInfo.ProgressState = "Indeterminate" }
|
|
'Normal' { $sync["Form"].taskbarItemInfo.ProgressState = "Normal" }
|
|
'Error' { $sync["Form"].taskbarItemInfo.ProgressState = "Error" }
|
|
'Paused' { $sync["Form"].taskbarItemInfo.ProgressState = "Paused" }
|
|
default { throw "[Set-WinUtilTaskbarItem] Invalid state" }
|
|
}
|
|
}
|
|
|
|
if ($overlay) {
|
|
switch ($overlay) {
|
|
'logo' {
|
|
$sync["Form"].taskbarItemInfo.Overlay = $sync["logorender"]
|
|
}
|
|
'checkmark' {
|
|
$sync["Form"].taskbarItemInfo.Overlay = $sync["checkmarkrender"]
|
|
}
|
|
'warning' {
|
|
$sync["Form"].taskbarItemInfo.Overlay = $sync["warningrender"]
|
|
}
|
|
'None' {
|
|
$sync["Form"].taskbarItemInfo.Overlay = $null
|
|
}
|
|
default {
|
|
if (Test-Path $overlay) {
|
|
$sync["Form"].taskbarItemInfo.Overlay = $overlay
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
if ($description) {
|
|
$sync["Form"].taskbarItemInfo.Description = $description
|
|
}
|
|
}
|