mirror of
https://github.com/ChrisTitusTech/winutil.git
synced 2024-11-14 22:55:52 -06:00
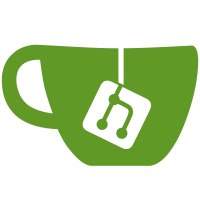
* Make 'ConvertTo-Icon' Private Function Parameter more stricter * Fix 'Invoke-WPFShortcut' Public Function Icon Support for the created shortcuts * Replace Tabs with Spaces to follow the conventions * Add new optional parameter to 'ConvertTo-Icon' and Improve the documentation for the function * Update the Description for 'ConvertTo-Icon' Function * Update some Documentation Wording in 'ConvertTo-Icon' Function * Change behavior of one case from throwing an exception to doing an early return Co-authored-by: Martin Wiethan <47688561+Marterich@users.noreply.github.com> * Update Examples for 'ConvertTo-Icon' Functions & Add a new Example, as well as some other changes Besides the updated documentation for 'ConvertTo-Icon' Function, the icon file path has changed from '$env:TEMP\cttlogo.ico' into '$env:LOCALAPPDATA\winutil\cttlogo.ico', and add edge-case of Folder not being found for the Icon File in 'ConvertTo-Icon' Code. --------- Co-authored-by: Martin Wiethan <47688561+Marterich@users.noreply.github.com>
94 lines
3.8 KiB
PowerShell
94 lines
3.8 KiB
PowerShell
function ConvertTo-Icon {
|
|
<#
|
|
|
|
.DESCRIPTION
|
|
This function will convert BMP, GIF, EXIF, JPG, PNG and TIFF to ICO file
|
|
|
|
.PARAMETER bitmapPath
|
|
The file path to bitmap image to make '.ico' file out of.
|
|
Supported file types according to Microsoft Documentation is the following:
|
|
BMP, GIF, EXIF, JPG, PNG and TIFF.
|
|
|
|
.PARAMETER iconPath
|
|
The file path to write the new '.ico' resource.
|
|
|
|
.PARAMETER overrideIconFile
|
|
An optional boolean Parameter that makes the function overrides
|
|
the Icon File Path if the file exists. Defaults to $true.
|
|
|
|
.EXAMPLE
|
|
try {
|
|
ConvertTo-Icon -bitmapPath "$env:TEMP\cttlogo.png" -iconPath "$env:TEMP\cttlogo.ico"
|
|
} catch [System.IO.FileNotFoundException] {
|
|
# Handle the thrown exception here...
|
|
}
|
|
|
|
This Example makes a '.ico' file at "$env:TEMP\cttlogo.ico" File Path using the bitmap file
|
|
found in "$env:TEMP\cttlogo.png", the function overrides the '.ico' File if it's found.
|
|
this function will throw a FileNotFound Exception at the event of not finding the provided bitmap File Path.
|
|
|
|
.EXAMPLE
|
|
try {
|
|
ConvertTo-Icon "$env:TEMP\cttlogo.png" "$env:TEMP\cttlogo.ico"
|
|
} catch [System.IO.FileNotFoundException] {
|
|
# Handle the thrown exception here...
|
|
}
|
|
|
|
This Example is the same as Example 1, but uses Positional Parameters instead.
|
|
|
|
.EXAMPLE
|
|
if (Test-Path "$env:TEMP\cttlogo.png") {
|
|
ConvertTo-Icon -bitmapPath "$env:TEMP\cttlogo.png" -iconPath "$env:TEMP\cttlogo.ico"
|
|
}
|
|
|
|
This Example is same as Example 1, but checks if the bitmap File exists before calling 'ConvertTo-Icon' Function.
|
|
This's the recommended way of using this function, as it doesn't require any try-catch blocks.
|
|
|
|
.EXAMPLE
|
|
try {
|
|
ConvertTo-Icon -bitmapPath "$env:TEMP\cttlogo.png" -iconPath "$env:TEMP\cttlogo.ico" -overrideIconFile $false
|
|
} catch [System.IO.FileNotFoundException] {
|
|
# Handle the thrown exception here...
|
|
}
|
|
|
|
This Example make use of '-overrideIconFile' Optional Parameter, the default for this paramter is $true.
|
|
By doing '-overrideIconFile $false', the 'ConvertTo-Icon' function will raise an exception that needs to be catched throw a 'catch' Code Block,
|
|
otherwise it'll crash the running PowerShell instance/process.
|
|
|
|
#>
|
|
param(
|
|
[Parameter(Mandatory=$true, position=0)]
|
|
[string]$bitmapPath,
|
|
[Parameter(Mandatory=$true, position=1)]
|
|
[string]$iconPath,
|
|
[Parameter(position=2)]
|
|
[bool]$overrideIconFile = $true
|
|
)
|
|
|
|
Add-Type -AssemblyName System.Drawing
|
|
|
|
if (Test-Path $bitmapPath) {
|
|
if ((Test-Path $iconPath) -AND ($overrideIconFile -eq $false)) {
|
|
Write-Host "[ConvertTo-Icon] Icon File is found at '$iconPath', and the 'overrideIconFile' Parameter is set to '$overrideIconFile'. Skipping the bitmap to icon convertion..." -ForegroundColor Yellow
|
|
return
|
|
}
|
|
|
|
# Load bitmap file into memory, and make an Icon version out of it
|
|
$b = [System.Drawing.Bitmap]::FromFile($bitmapPath)
|
|
$icon = [System.Drawing.Icon]::FromHandle($b.GetHicon())
|
|
|
|
# Create the folder for the new icon file if it doesn't exists
|
|
$iconFolder = (New-Object System.IO.FileInfo($iconPath)).Directory.FullName
|
|
[System.IO.Directory]::CreateDirectory($iconFolder) | Out-Null
|
|
|
|
# Write the Icon File and do some cleaning-up
|
|
$file = New-Object System.IO.FileStream($iconPath, 'OpenOrCreate')
|
|
$icon.Save($file)
|
|
$file.Close()
|
|
$icon.Dispose()
|
|
}
|
|
else {
|
|
throw [System.IO.FileNotFoundException] "[ConvertTo-Icon] The provided bitmap File Path is not found at '$bitmapPath'."
|
|
}
|
|
}
|