mirror of
https://github.com/ChrisTitusTech/winutil.git
synced 2025-07-15 09:28:46 -05:00
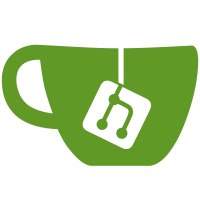
* Add right click context menu for app entries with install, uninstall, and info options * Add hand cursor on mouse over for button in inputXML.xaml
121 lines
5.9 KiB
PowerShell
121 lines
5.9 KiB
PowerShell
function Initialize-WPFUI {
|
|
[OutputType([void])]
|
|
param(
|
|
[Parameter(Mandatory)]
|
|
[string]$TargetGridName
|
|
)
|
|
|
|
switch ($TargetGridName) {
|
|
"appscategory"{
|
|
# TODO
|
|
# Switch UI generation of the sidebar to this function
|
|
# $sync.ItemsControl = Initialize-InstallAppArea -TargetElement $TargetGridName
|
|
# ...
|
|
|
|
# Create and configure a popup for displaying selected apps
|
|
$selectedAppsPopup = New-Object Windows.Controls.Primitives.Popup
|
|
$selectedAppsPopup.IsOpen = $false
|
|
$selectedAppsPopup.PlacementTarget = $sync.WPFselectedAppsButton
|
|
$selectedAppsPopup.Placement = [System.Windows.Controls.Primitives.PlacementMode]::Bottom
|
|
$selectedAppsPopup.AllowsTransparency = $true
|
|
|
|
# Style the popup with a border and background
|
|
$selectedAppsBorder = New-Object Windows.Controls.Border
|
|
$selectedAppsBorder.SetResourceReference([Windows.Controls.Control]::BackgroundProperty, "MainBackgroundColor")
|
|
$selectedAppsBorder.SetResourceReference([Windows.Controls.Control]::BorderBrushProperty, "MainForegroundColor")
|
|
$selectedAppsBorder.SetResourceReference([Windows.Controls.Control]::BorderThicknessProperty, "ButtonBorderThickness")
|
|
$selectedAppsBorder.Width = 200
|
|
$selectedAppsBorder.Padding = 5
|
|
$selectedAppsPopup.Child = $selectedAppsBorder
|
|
$sync.selectedAppsPopup = $selectedAppsPopup
|
|
|
|
# Add a stack panel inside the popup's border to organize its child elements
|
|
$sync.selectedAppsstackPanel = New-Object Windows.Controls.StackPanel
|
|
$selectedAppsBorder.Child = $sync.selectedAppsstackPanel
|
|
|
|
# Close selectedAppsPopup when mouse leaves both button and selectedAppsPopup
|
|
$sync.WPFselectedAppsButton.Add_MouseLeave({
|
|
if (-not $sync.selectedAppsPopup.IsMouseOver) {
|
|
$sync.selectedAppsPopup.IsOpen = $false
|
|
}
|
|
})
|
|
$selectedAppsPopup.Add_MouseLeave({
|
|
if (-not $sync.WPFselectedAppsButton.IsMouseOver) {
|
|
$sync.selectedAppsPopup.IsOpen = $false
|
|
}
|
|
})
|
|
|
|
# Creates the popup that is displayed when the user right-clicks on an app entry
|
|
# This popup contains buttons for installing, uninstalling, and viewing app information
|
|
|
|
$appPopup = New-Object Windows.Controls.Primitives.Popup
|
|
$appPopup.StaysOpen = $false
|
|
$appPopup.Placement = [System.Windows.Controls.Primitives.PlacementMode]::Bottom
|
|
$appPopup.AllowsTransparency = $true
|
|
# Store the popup globally so the position can be set later
|
|
$sync.appPopup = $appPopup
|
|
|
|
$appPopupStackPanel = New-Object Windows.Controls.StackPanel
|
|
$appPopupStackPanel.Orientation = "Horizontal"
|
|
$appPopupStackPanel.Add_MouseLeave({
|
|
$sync.appPopup.IsOpen = $false
|
|
})
|
|
$appPopup.Child = $appPopupStackPanel
|
|
|
|
$appButtons = @(
|
|
[PSCustomObject]@{ Name = "Install"; Icon = [char]0xE118 },
|
|
[PSCustomObject]@{ Name = "Uninstall"; Icon = [char]0xE74D },
|
|
[PSCustomObject]@{ Name = "Info"; Icon = [char]0xE946 }
|
|
)
|
|
foreach ($button in $appButtons) {
|
|
$newButton = New-Object Windows.Controls.Button
|
|
$newButton.Style = $sync.Form.Resources.AppEntryButtonStyle
|
|
$newButton.Content = $button.Icon
|
|
$appPopupStackPanel.Children.Add($newButton) | Out-Null
|
|
|
|
# Dynamically load the selected app object so the buttons can be reused and do not need to be created for each app
|
|
switch ($button.Name) {
|
|
"Install" {
|
|
$newButton.Add_MouseEnter({
|
|
$appObject = $sync.configs.applicationsHashtable.$($sync.appPopupSelectedApp)
|
|
$this.ToolTip = "Install or Upgrade $($appObject.content)"
|
|
})
|
|
$newButton.Add_Click({
|
|
$appObject = $sync.configs.applicationsHashtable.$($sync.appPopupSelectedApp)
|
|
Invoke-WPFInstall -PackagesToInstall $appObject
|
|
})
|
|
}
|
|
"Uninstall" {
|
|
$newButton.Add_MouseEnter({
|
|
$appObject = $sync.configs.applicationsHashtable.$($sync.appPopupSelectedApp)
|
|
$this.ToolTip = "Uninstall $($appObject.content)"
|
|
})
|
|
$newButton.Add_Click({
|
|
$appObject = $sync.configs.applicationsHashtable.$($sync.appPopupSelectedApp)
|
|
Invoke-WPFUnInstall -PackagesToUninstall $appObject
|
|
})
|
|
}
|
|
"Info" {
|
|
$newButton.Add_MouseEnter({
|
|
$appObject = $sync.configs.applicationsHashtable.$($sync.appPopupSelectedApp)
|
|
$this.ToolTip = "Open the application's website in your default browser`n$($appObject.link)"
|
|
})
|
|
$newButton.Add_Click({
|
|
$appObject = $sync.configs.applicationsHashtable.$($sync.appPopupSelectedApp)
|
|
Start-Process $appObject.link
|
|
})
|
|
}
|
|
}
|
|
}
|
|
}
|
|
"appspanel" {
|
|
$sync.ItemsControl = Initialize-InstallAppArea -TargetElement $TargetGridName
|
|
Initialize-InstallCategoryAppList -TargetElement $sync.ItemsControl -Apps $sync.configs.applicationsHashtable
|
|
}
|
|
default {
|
|
Write-Output "$TargetGridName not yet implemented"
|
|
}
|
|
}
|
|
}
|
|
|