mirror of
https://github.com/ChrisTitusTech/winutil.git
synced 2024-11-15 07:05:51 -06:00
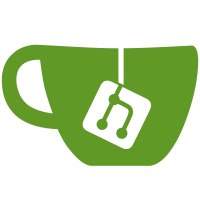
* Replace Tabs with Spaces to follow the conventions
* Add Preprocessing in Compiler
* Compile from Anywhere you want - Running 'Compile.ps1' Works in any directory you call it from
* Code Formatting Changes
* Result of Preprocessing Step in 'Compile.ps1' Script - Remove Trailing Whitespace Characters
* Make Preprocessing more advanced
* Move Preprocessing to a separate script file
* Make Self Modification impossible for 'tools/Do-PreProcessing.ps1' Script - Make the workingdir same as sync.PSScriptRoot for consistency
* Revert commit b5dffd671f
* Patched a Bug of some Excluded Files not actually get excluded in 'Get-ChildItem' PS Cmdlet
* Update Replace Regex for Code Formatting in 'Do-PreProcessing' Script Tool
* Rename 'Do-PreProcessing' to 'Invoke-Preprocessing' - Update some Comments
* Make 'Invoke-Preprocessing' Modular - Update RegEx to handle more cases - Update Documentation - Add Validations & Useful feedback upon error
* Replace Tabs with Spaces to follow the conventions - 'applications.json' File
* Code Formatting Changes - 'Copy-Files' Private Function
* Update Replace Regex for Code Formatting in 'Invoke-Preprocessing' Script Tool
* Replace Tabs with Spaces to follow the conventions - Make 'ExcludedFiles' validation step check all filepaths before finally checking if any has failed
* Result of 'Invoke-Preprocessing' Script
* Update Replace Regex for Code Formatting in 'Invoke-Preprocessing' Script Tool
87 lines
3.1 KiB
PowerShell
87 lines
3.1 KiB
PowerShell
function Set-WinUtilTaskbaritem {
|
|
<#
|
|
|
|
.SYNOPSIS
|
|
Modifies the Taskbaritem of the WPF Form
|
|
|
|
.PARAMETER value
|
|
Value can be between 0 and 1, 0 being no progress done yet and 1 being fully completed
|
|
Value does not affect item without setting the state to 'Normal', 'Error' or 'Paused'
|
|
Set-WinUtilTaskbaritem -value 0.5
|
|
|
|
.PARAMETER state
|
|
State can be 'None' > No progress, 'Indeterminate' > inf. loading gray, 'Normal' > Gray, 'Error' > Red, 'Paused' > Yellow
|
|
no value needed:
|
|
- Set-WinUtilTaskbaritem -state "None"
|
|
- Set-WinUtilTaskbaritem -state "Indeterminate"
|
|
value needed:
|
|
- Set-WinUtilTaskbaritem -state "Error"
|
|
- Set-WinUtilTaskbaritem -state "Normal"
|
|
- Set-WinUtilTaskbaritem -state "Paused"
|
|
|
|
.PARAMETER overlay
|
|
Overlay icon to display on the taskbar item, there are the presets 'None', 'logo' and 'checkmark' or you can specify a path/link to an image file.
|
|
CTT logo preset:
|
|
- Set-WinUtilTaskbaritem -overlay "logo"
|
|
Checkmark preset:
|
|
- Set-WinUtilTaskbaritem -overlay "checkmark"
|
|
Warning preset:
|
|
- Set-WinUtilTaskbaritem -overlay "warning"
|
|
No overlay:
|
|
- Set-WinUtilTaskbaritem -overlay "None"
|
|
Custom icon (needs to be supported by WPF):
|
|
- Set-WinUtilTaskbaritem -overlay "C:\path\to\icon.png"
|
|
|
|
.PARAMETER description
|
|
Description to display on the taskbar item preview
|
|
Set-WinUtilTaskbaritem -description "This is a description"
|
|
#>
|
|
param (
|
|
[string]$state,
|
|
[double]$value,
|
|
[string]$overlay,
|
|
[string]$description
|
|
)
|
|
|
|
if ($value) {
|
|
$sync["Form"].taskbarItemInfo.ProgressValue = $value
|
|
}
|
|
|
|
if ($state) {
|
|
switch ($state) {
|
|
'None' { $sync["Form"].taskbarItemInfo.ProgressState = "None" }
|
|
'Indeterminate' { $sync["Form"].taskbarItemInfo.ProgressState = "Indeterminate" }
|
|
'Normal' { $sync["Form"].taskbarItemInfo.ProgressState = "Normal" }
|
|
'Error' { $sync["Form"].taskbarItemInfo.ProgressState = "Error" }
|
|
'Paused' { $sync["Form"].taskbarItemInfo.ProgressState = "Paused" }
|
|
default { throw "[Set-WinUtilTaskbarItem] Invalid state" }
|
|
}
|
|
}
|
|
|
|
if ($overlay) {
|
|
switch ($overlay) {
|
|
'logo' {
|
|
$sync["Form"].taskbarItemInfo.Overlay = "$env:LOCALAPPDATA\winutil\cttlogo.png"
|
|
}
|
|
'checkmark' {
|
|
$sync["Form"].taskbarItemInfo.Overlay = "$env:LOCALAPPDATA\winutil\checkmark.png"
|
|
}
|
|
'warning' {
|
|
$sync["Form"].taskbarItemInfo.Overlay = "$env:LOCALAPPDATA\winutil\warning.png"
|
|
}
|
|
'None' {
|
|
$sync["Form"].taskbarItemInfo.Overlay = $null
|
|
}
|
|
default {
|
|
if (Test-Path $overlay) {
|
|
$sync["Form"].taskbarItemInfo.Overlay = $overlay
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
if ($description) {
|
|
$sync["Form"].taskbarItemInfo.Description = $description
|
|
}
|
|
}
|